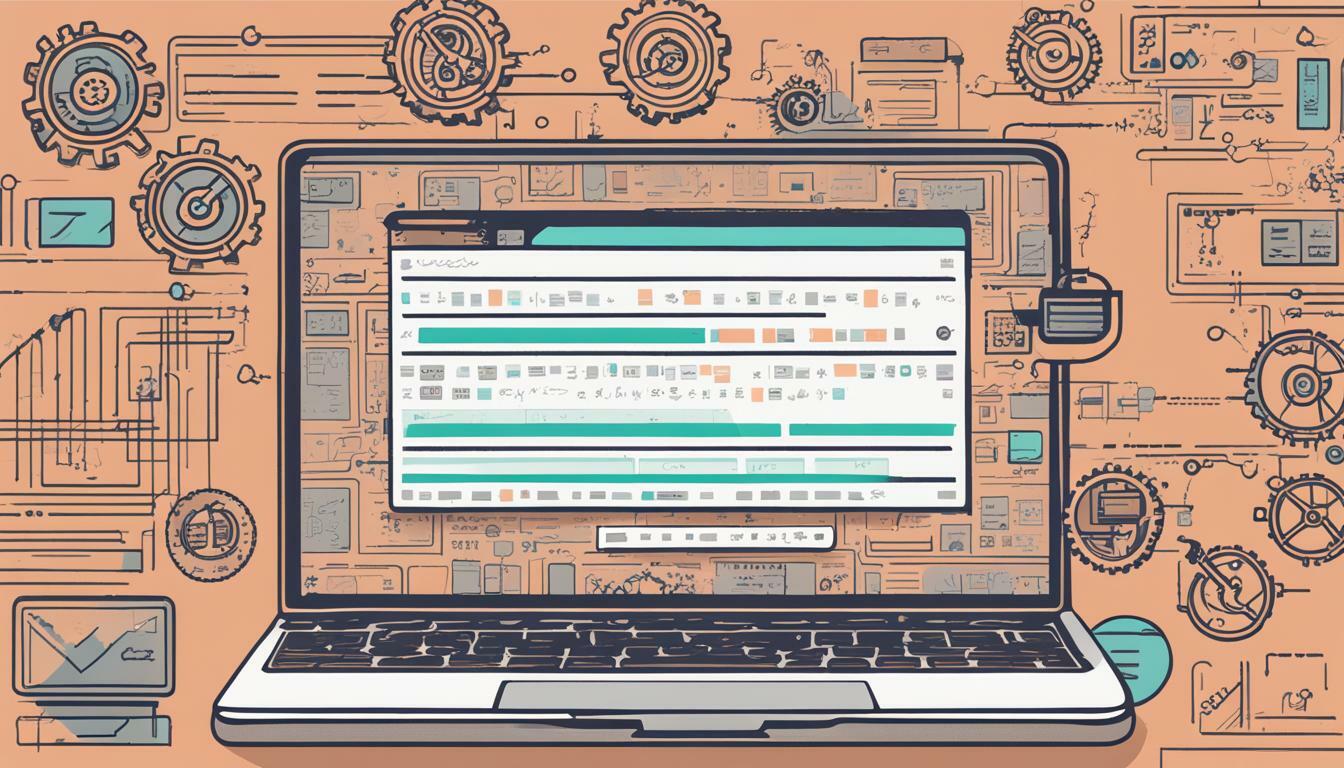
Welcome to our guide on JavaScript code snippets, examples, and best practices for efficient coding. As a developer, you know the importance of writing clean, organized, and efficient code to ensure the success of your projects. But with the constantly evolving technology in the digital world, it can be challenging to stay up-to-date with the latest coding techniques and best practices. That’s where JavaScript code snippets come in.
JavaScript code snippets are small blocks of pre-written code that can be easily integrated into your web applications to simplify and speed up your coding process. They can help you accomplish specific tasks more efficiently and effectively, whether you’re working on form validation, DOM manipulation, event handling, or other common coding tasks. By using JavaScript examples and code samples, you can streamline your development process and improve the overall quality of your code.
Importance of JavaScript Code Snippets
JavaScript coding can be a complex and time-consuming process. Fortunately, by utilizing JavaScript code snippets, developers can streamline their workflow and improve their productivity. In this section, we will highlight the importance of JavaScript code snippets and provide some valuable JavaScript coding tips and techniques that can help you become a more efficient coder.
Firstly, JavaScript code snippets can significantly improve code readability. By breaking down complex tasks into smaller, more manageable pieces, code snippets can make your code easier to understand and maintain. This can save time and effort in the long run, as developers can more quickly and easily debug and modify their code.
Another advantage of using JavaScript code snippets is that they can encourage best practices in JavaScript coding. By leveraging pre-existing code examples, developers can ensure that they are following industry-standard approaches and avoiding common pitfalls and mistakes. This can lead to more stable and reliable code.
Lastly, JavaScript code snippets can improve productivity by simplifying the coding process. By utilizing pre-existing snippets for common tasks, such as form validation or event handling, developers can save time and avoid redundant coding. This allows them to focus on more complex and innovative aspects of their projects.
By following these JavaScript coding tips and techniques, developers can take full advantage of JavaScript code snippets and become more efficient coders.
Using JavaScript Code Snippets Effectively
Incorporating JavaScript code examples can greatly enhance your coding process. Here are some tips to help you use snippets more effectively:
1. Integrate Snippets into Your Codebase
Instead of reinventing the wheel every time you encounter a common coding problem, consider incorporating a JavaScript code snippet into your codebase. This can save you time and effort in the long run.
2. Modify Snippets to Suit Your Needs
Don’t be afraid to modify code snippets to fit your specific project requirements. You can tweak variables, parameters, and functions to better suit your needs. Just ensure that you thoroughly test any modifications to ensure the code still performs as intended.
3. Leverage Snippets for Faster Development
JavaScript code snippets can be particularly useful when working on tight deadlines or small projects. By utilizing existing code, you can more quickly develop and deploy your application.
4. Organize Snippets for Easy Access
Ensure that your code snippets are organized and easily accessible. This can be achieved by utilizing code repositories like GitHub or creating your own internal library of snippets. This can help you quickly find the code you need when you need it.
By following these tips, you can more effectively implement JavaScript code snippets into your coding process.
JavaScript Code Snippets for Common Tasks
JavaScript code implementation can be streamlined by utilizing code snippets that are commonly used for various tasks. Below are some examples and explanations of tasks that can be simplified and expedited with code snippets.
Form Validation
Form validation is an essential task for any website that collects user input. By implementing JavaScript code snippets, you can ensure that your forms are filled out correctly before being submitted. One popular technique is to use regular expressions to validate email addresses, phone numbers, and other input types.
Code Snippet | Description |
---|---|
function validateForm() { var x = document.forms[“myForm”][“email”].value; if (x == “”) { alert(“Email must be filled out”); return false; } } | This code snippet validates if the email input is empty, and if so, it alerts the user and prevents the form from being submitted. |
DOM Manipulation
DOM manipulation is used to dynamically update the content and style of web pages. JavaScript code snippets can make these updates faster and more efficient. One common use case is to change the background color of an element based on user input or events.
Code Snippet | Description |
---|---|
function changeColor() { document.getElementById(“myDiv”).style.backgroundColor = “red”; } | This code snippet changes the background color of the element with the ID “myDiv” to red. |
Event Handling
Event handling is a crucial aspect of modern web development, as it enables developers to respond to user interactions and other events. JavaScript code snippets can simplify the implementation of event listeners and handlers, allowing for more efficient development.
Code Snippet | Description |
---|---|
document.getElementById(“myButton”).addEventListener(“click”, function() { alert(“Button clicked!”); }); | This code snippet adds a listener to the element with the ID “myButton” that triggers an alert when clicked. |
By implementing these and other JavaScript code snippets, you can significantly improve the efficiency and quality of your code. JavaScript code implementation can be made easier with these snippets, helping you to achieve faster development and better results.
Best Practices for JavaScript Code Snippets
When using JavaScript code snippets, it is important to adhere to best practices to ensure clean, maintainable, and efficient code. Here are some key best practices to keep in mind:
- Code organization: Keep your code organized and easy to read by dividing it into logical sections and using consistent formatting.
- Commenting: Use comments to explain code functionality and help other developers understand your code.
- Variable naming conventions: Use descriptive and meaningful names for variables and avoid abbreviations.
- Cross-browser compatibility: Test your code in multiple browsers to ensure compatibility.
By following these best practices, you can write code that is not only functional but also easy to maintain and understand for yourself and others.
Advanced JavaScript Code Snippets
Once you’ve got a good handle on basic JavaScript coding techniques, it’s time to level up your skills with advanced code snippets. These tips are geared towards experienced developers looking to take their projects to the next level.
Closures
Closures are a powerful technique in JavaScript that allow for the creation of private variables and functions. By wrapping functions in an outer function, you can create a “private” scope that is inaccessible from outside code. This can be useful for encapsulating logic and creating modular code.
Example:
function outer() { var privateVar = "I'm private"; function inner() { console.log(privateVar); } return inner; } var closure = outer(); closure();
In this example, the outer function creates a private variable “privateVar” and a function “inner” that has access to it. The function is then returned and assigned to the “closure” variable, which can now be called to access the private variable through the “inner” function.
Object-Oriented Programming
JavaScript is a versatile language that can be used for object-oriented programming. Using constructor functions and prototypes, you can create classes and objects with properties and methods, just like in other object-oriented languages.
Example:
function Person(name, age) { this.name = name; this.age = age; } Person.prototype.sayHello = function() { console.log("Hello, my name is " + this.name); }; var person = new Person("Alice", 25); person.sayHello();
In this example, the “Person” constructor function creates a new object with properties “name” and “age”. The “sayHello” method is added to the “Person” prototype, which means it is accessible to all objects created from the “Person” constructor. Finally, a new object “person” is created from the “Person” constructor and the “sayHello” method is called on it.
Asynchronous Programming
Asynchronous programming in JavaScript allows for non-blocking code that can handle multiple tasks at once. This is achieved through techniques like callbacks, promises, and async/await.
Example:
function fetchData(callback) { setTimeout(function() { callback("Data fetched successfully"); }, 2000); } fetchData(function(data) { console.log(data); });
In this example, the “fetchData” function simulates fetching data from an external source by waiting for 2 seconds before calling the provided callback function with the message “Data fetched successfully”. The “fetchData” function is then called with a callback function that logs the returned message.
By utilizing these advanced JavaScript code snippets, you can take your coding skills to the next level and build even more powerful applications.
JavaScript Code Snippets for Performance Optimization
Optimizing the performance of your JavaScript code is crucial for ensuring smooth and efficient applications. Here are some useful tips and techniques to help you achieve better results:
Optimize Loops
Loops are essential for iterating over arrays and performing repetitive tasks, but they can also be a major bottleneck in your code. To optimize loops, consider using built-in array methods like forEach(), map(), and filter() instead of traditional for loops. These methods are optimized for performance and can often result in faster and more efficient code.
Reduce Memory Usage
Excessive memory usage can slow down your application and even lead to crashes. To minimize memory usage, be mindful of creating unnecessary variables or objects, and always remove references to objects when they are no longer needed. Additionally, consider using object pooling or memoization techniques to reuse objects and reduce memory allocation.
Leverage Caching
Caching can greatly improve the performance of your application by reducing the amount of data that needs to be retrieved or processed. Consider caching frequently used data in memory or on the client-side, and using browser caching for static assets like images and scripts.
Utilize Browser APIs Effectively
Modern browsers offer a wealth of APIs for enhancing performance, such as the Web Workers API for multi-threaded processing, the requestAnimationFrame() method for optimized animations, and the localStorage API for persistent storage of data. Be sure to take advantage of these tools to optimize your code and improve user experience.
By following these JavaScript coding tips and techniques, you can greatly improve the performance of your applications and provide a better experience for your users.
Conclusion
JavaScript code snippets are a valuable tool for any developer looking to streamline their coding process. By utilizing these code examples, you can enhance productivity, improve code readability, and encourage best practices in JavaScript coding. Best of all, there are plenty of resources available online for developers to find and integrate these code snippets into their projects.
Throughout this article, we have discussed the importance of using JavaScript code snippets, how to effectively implement them into projects, and showcased specific snippets for common tasks. We have also covered best practices for using JavaScript snippets, advanced concepts, and snippets for performance optimization.
By following these tips and utilizing JavaScript code snippets, you can ensure that your code is clean, maintainable, and efficient. So the next time you’re working on a JavaScript project, be sure to consider using code snippets to simplify your development process. Happy coding!